Coding Exercise – Three Sum Closest
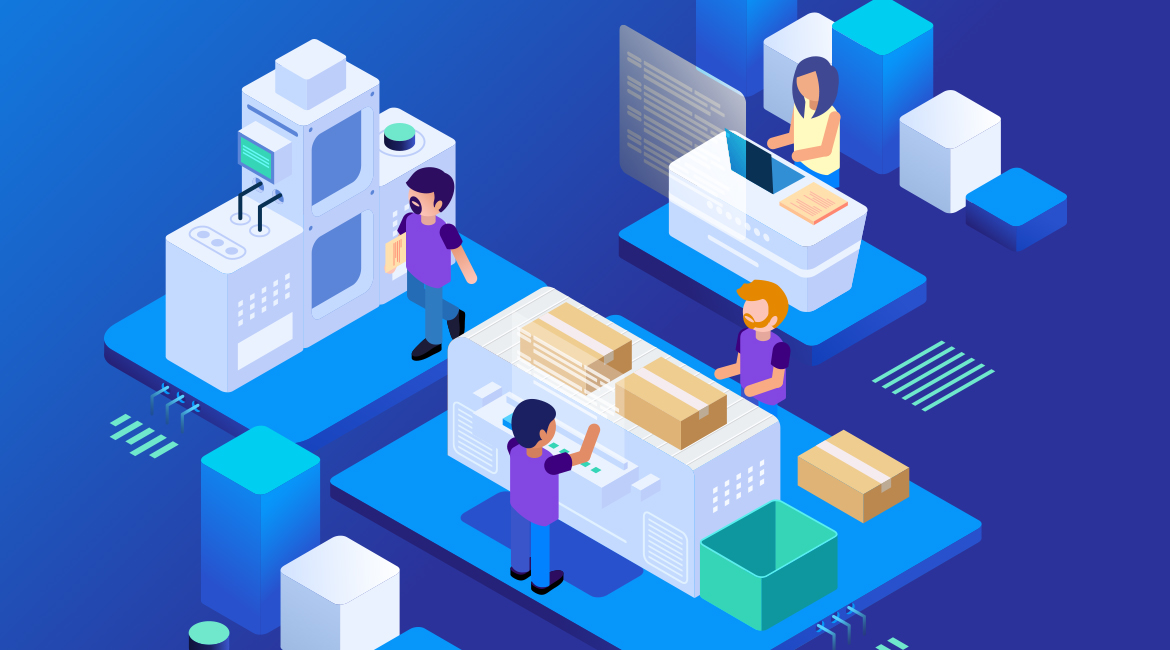
Three Sum Closest – for this coding exercise, we’re going to solve the following:
- Given an array nums of n integers and an integer target
-
Find three integers in nums such that the sum is closest to target.
- Return the sum of the three integers.
- You may assume that each input would have exactly one solution.
Example:
-
Input: nums = [-1,2,1,-4], target = 1
- Output: 2
Explanation:The sum that is closest to the target is 2. (-1 + 2 + 1 = 2).Constraints:3 <= nums.length <= 10^3-10^3 <= nums[i] <= 10^3-10^4 <= target <= 10^4
Solution in – SWIFT
class Platini {
func threeSumClosest(_ nums: [Int], _ target: Int) -> Int {
let sortedNums = nums.sorted()
var result = nums[0] + nums[1] + nums[2]
var diff = abs(result - target)
for (index, num) in sortedNums.enumerated() {
var start = index + 1
var end = sortedNums.count - 1
while start < end {
var sum = num + sortedNums[start] + sortedNums[end]
guard sum != target else {
return target
}
let newDiff = abs(sum - target)
if newDiff < diff { diff = newDiff result = sum } if sum > target {
end -= 1
} else {
start += 1
}
}
}
return result
}
}
Solution in – C#
public class Platini {
private int Search(int[] nums, int start, int target) {
int i = start;
int j = nums.Length - 1;
int res = nums[i] + nums[j];
while (i < j) {
int num = nums[i] + nums[j];
if (Math.Abs(num - target) < Math.Abs(res - target)) res = num;
if (num == target) break;
if (num < target) {
i += 1;
} else {
j -= 1;
}
}
return res;
}
public int ThreeSumClosest(int[] nums, int target) {
Array.Sort(nums);
int res = nums[0] + nums[1] + nums[2];
for (int i = 0; i < nums.Length - 2; i += 1) {
int sum = nums[i] + Search(nums, i + 1, target - nums[i]);
if (Math.Abs(sum - target) < Math.Abs(res - target)) res = sum;
}
return res;
}
}
Solution in – JavaScript
/**
* @param {number[]} nums
* @param {number} target
* @return {number}
*/
var threeSumClosest = function(nums, target) {
nums.sort((n1, n2) => n1 - n2)
let min = Infinity
const search = (i, j, base) => {
while (i < j) {
const sum = base + nums[i] + nums[j]
if (Math.abs(target - sum) < Math.abs(target - min)) min = sum if (min === target) break if (sum > target) {
j -= 1
} else {
i += 1
}
}
}
for (let i = 0; i < nums.length; i += 1) {
if (nums[i] === nums[i - 1]) continue
search(i + 1, nums.length - 1, nums[i])
if (min === target) break
}
return min
}
Solution in – Ruby
# @param {Integer[]} nums
# @param {Integer} target
# @return {Integer}
def three_sum_closest(nums, target)
nums.sort!
result = nums[0] + nums[1] + nums[2]
return result unless result != target
nums.each_with_index { |num, index|
i = index + 1
j = nums.size - 1
while i < j do
sum = num + nums[i] + nums[j]
if (target - sum).abs < (target - result).abs then result = sum end if sum > target then
j -= 1
while j > i && nums[j] == nums[j + 1] do
j -= 1
end
elsif sum < target then i += 1 while j > i && nums[i] == nums[i - 1] do
i += 1
end
else
return result
end
end
}
return result
end