Coding Exercise – Sum of Two
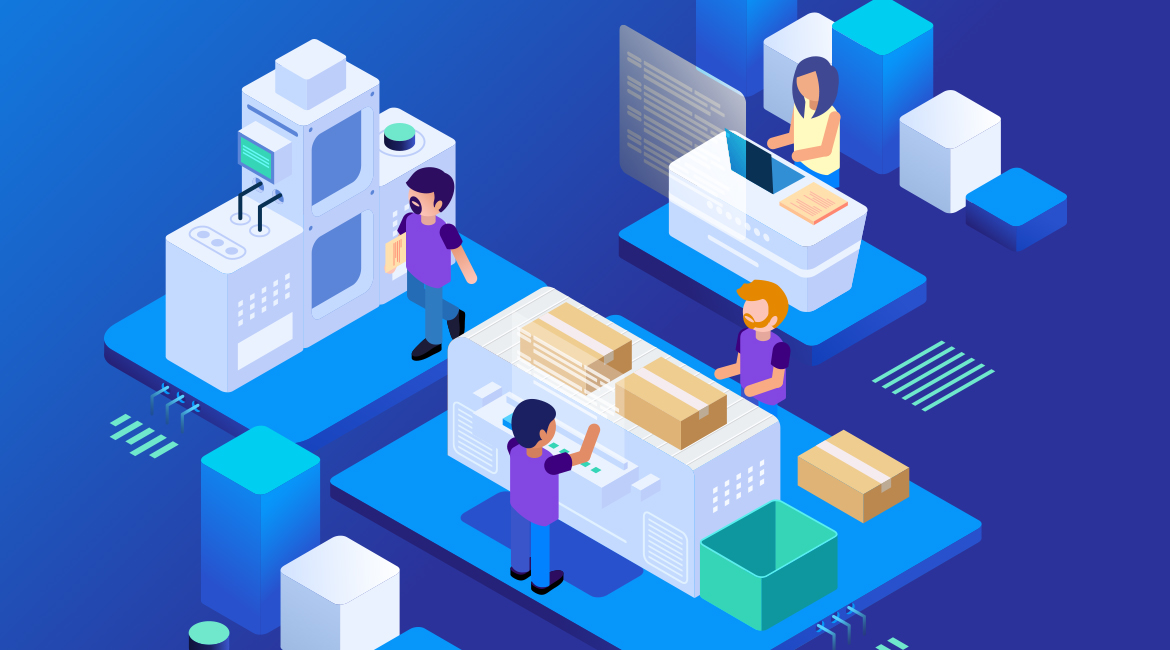
Sum of Two – for this coding exercise, we’re going to solve the following:
- Given an array of integers, return indices of the two numbers such that they add up to a specific target.
- You may assume that each input would have exactly one solution, and you may not use the same element twice.
Example:
- Given nums = [2, 7, 11, 15], target = 9,
- Because nums[0] + nums[1] = 2 + 7 = 9,
- Outpu: [2,7]
-
Explanation: The sum of 2 and 7 is 9. Therefore index1 = 1, index2 = 2.
Solution in – SWIFT
class Platini {
func SumTwoNumbers(_ nums: [Int], _ target: Int) -> [Int] {
var dict = Dictionary<Int, Array<Int>>()
for (index, value) in nums.enumerated() {
var arr = dict[value, default: []]
arr.append(index)
dict[value] = arr
}
for (index, value) in nums.enumerated() {
var remainder = target - value
guard let arr = dict[remainder] else {
continue
}
let result = arr.filter { i in i != index }
if result.count > 0 {
return [index, result[0]]
}
}
return [Int]()
}
}
Solution in – C#
public class Epic {
public int[] SumTwoNumbers(int[] numbers, int target) {
int i = 0;
int j = numbers.Length - 1;
while (i < j) { int sum = numbers[i] + numbers[j];
if (sum == target)
return new int[]{ i + 1, j + 1 };
if (sum > target) {
j -= 1;
} else {
i += 1;
}
}
return new int[0];
}
}
Solution in – JavaScript
/**
* @param {number[]} nums
* @param {number} target
* @return {number[]}
*/
var twoSumNumbers = function(nums, target) {
var set = new Set(nums);
var indexA = null; var indexB = null;
for (let i = 0; i < nums.length; i += 1) {
var numberA = nums[i];
var numberB = target - numberA;
if (set.has(numberB)) {
var index = nums.indexOf(numberB);
if (i === index) continue;
indexA = i;
indexB = index;
break;
}
}
if (indexA === null) throw new Error('Error');
return [indexA, indexB];
};
Solution in – Ruby
# @param {Integer[]} nums
# @param {Integer} target
# @return {Integer[]}
def twoSumNumbers(nums, target)
nums.each_with_index do |num, index|
remain = target - num
i = nums.index { |tmp| tmp == remain }
if i && i != index then
break [index, i]
end
end
end