Coding Exercise – Add Two Numbers
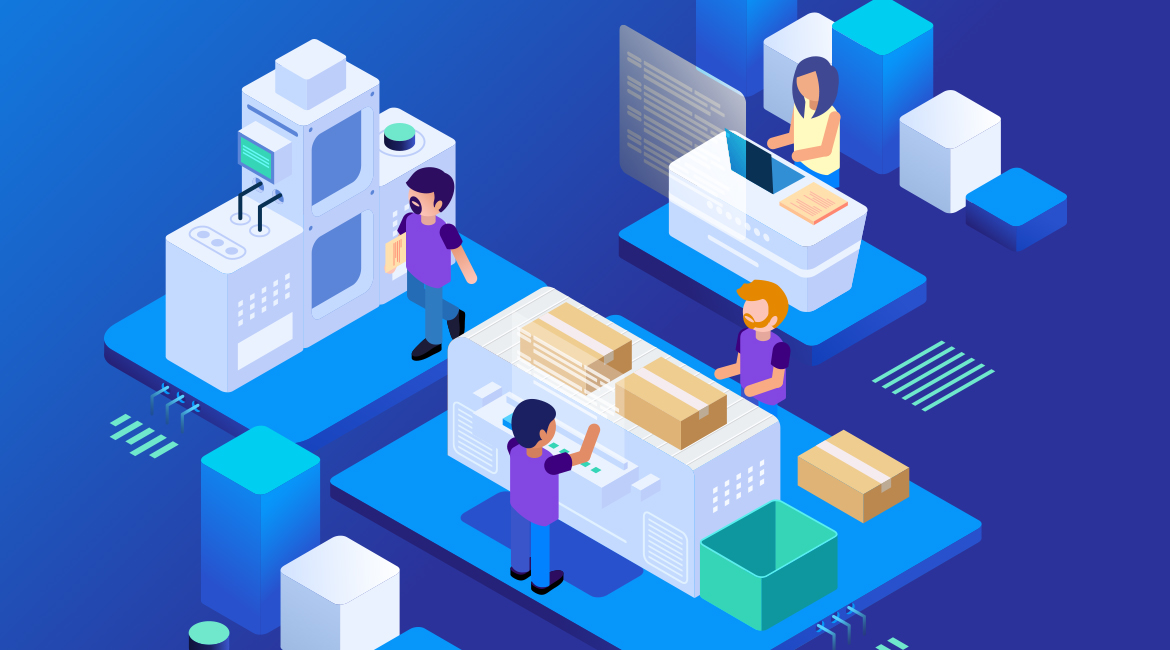
Add Two Numbers – for this coding exercise, we’re going to solve the following:
- Given two non-empty linked lists representing two non-negative integers.
-
The digits are stored in reverse order and each of their nodes contain a single digit.
- Add the two numbers and return it as a linked list.
- You may assume the two numbers do not contain any leading zero, except the number 0 itself.
Example:
-
Input: (2 -> 4 -> 3) + (5 -> 6 -> 4)
- Output: 7 -> 0 -> 8
Solution in – SWIFT
/**
* Definition for singly-linked list.
*/
public class ListNode {
public var val: Int
public var next: ListNode?
public init(_ val: Int) {
self.val = val
self.next = nil
}
}
class Platini {
func addTwoNumbers(_ l1: ListNode?, _ l2: ListNode?) -> ListNode? {
var result: ListNode! = nil
var node: ListNode! = nil
var remainder: Int = 0
var list1 = l1
var list2 = l2
while list1 != nil || list2 != nil {
let sum = (list1?.val ?? 0) + (list2?.val ?? 0) + remainder
let num = sum % 10
remainder = sum / 10
let newNode = ListNode(num)
if result == nil {
result = newNode
node = newNode
} else {
node.next = newNode
node = newNode
}
list1 = list1?.next
list2 = list2?.next
}
if remainder > 0 {
node.next = ListNode(remainder)
}
return result
}
}
Solution in – C#
public class ListNode {
public int val;
public ListNode next;
public ListNode(int x) { val = x; }
}
public class Platini {
public ListNode AddTwoNumbers(ListNode l1, ListNode l2) {
int remain = 0;
ListNode res = new ListNode(0);
ListNode cur = res;
while (l1 != null || l2 != null) {
int num = (l1 == null ? 0 : l1.val) + (l2 == null ? 0 : l2.val) + remain;
cur.next = new ListNode(num % 10);
remain = num >= 10 ? 1 : 0;
cur = cur.next;
if (l1 != null) l1 = l1.next;
if (l2 != null) l2 = l2.next;
}
if (remain > 0) cur.next = new ListNode(1);
return res.next;
}
}
Solution in – JavaScript
/**
* Definition for singly-linked list.
*/
function ListNode(val) {
this.val = val;
this.next = null;
}
/**
* @param {ListNode} l1
* @param {ListNode} l2
* @return {ListNode}
*/
var addTwoNumbers = function(l1, l2) {
const result = new ListNode()
let head = result
let remain = 0
while (l1 || l2) {
const sum = (l1 ? l1.val : 0) + (l2 ? l2.val : 0) + remain
const val = sum % 10
remain = sum >= 10 ? 1 : 0
head.next = new ListNode(val)
head = head.next
l1 = l1 ? l1.next : null
l2 = l2 ? l2.next : null
}
if (remain) head.next = new ListNode(remain)
return result.next
}
Solution in – Ruby
# Definition for singly-linked list.
class ListNode
attr_accessor :val, :next
def initialize(val)
@val = val
@next = nil
end
end
# @param {ListNode} l1
# @param {ListNode} l2
# @return {ListNode}
def addTwoNumbers(l1, l2)
carry = 0
head = nil
cur = nil
while l1 || l2
sum = (l1 ? l1.val : 0) + (l2 ? l2.val : 0) + carry
carry = sum.div(10)
val = sum % 10
node = ListNode.new(val)
if !head
head = node
cur = head
else
cur.next = node
cur = node
end
if l1
l1 = l1.next
end
if l2
l2 = l2.next
end
end
if carry != 0
cur.next = ListNode.new(carry)
end
return head
end